When you automate browser applications there is no way around an automation tool as Selenium. It is optimized for browser interaction and executes blazingly fast.
However sometimes you need to leave the confines of your browser to interact with another application or your operating system. This is simply not possible with Selenium alone.
AskUI shines in this regard as it is not limited to a browser with its visual selectors and real input commands like keypresses and mouse movement.
In this post you will learn to setup Selenium together with AskUI to define workflows that can leave the browser.
Prerequisites
Install Selenium
The first thing you have to do is to install the selenium-webdriver. And since we are using TypeScript, let's install the type definitions also:
Configure Selenium
With Selenium ready to go, you have to head over to the file helpers/askui-helpers.ts, configure the Selenium Webdriver there. Here is how the the content of the file should look like:
- Import the necessary classes to initialize a
WebDriver
for the Chrome browser - Initialize the
WebDriver
for Chrome browser - Make sure the browser gets closed after execution
- Export the
WebDriver
for use in a AskUI Workflow
Write a Sample AskUI Workflow
With the WebDriver set up, hop over to the file my-first-askui-test-seuite.test.ts and import it there alongside a few more classes you need to write Selenium code. Add this to the start of the file:
Now you can implement a very simple workflow:
- Maximize the browser window
- Open the website https://google.com
- Dismiss the cookie popup by clicking the Reject all button
- Search for webdriver and wait for the search results to appear
- Then with AskUI: Click on the button with text Images to get to the image search
- Then refine your search by clicking on architecture
- Move your mouse to the image where a Chrome and a Firefox logo in it
Testrun
The following gif shows the execution of the whole workflow:
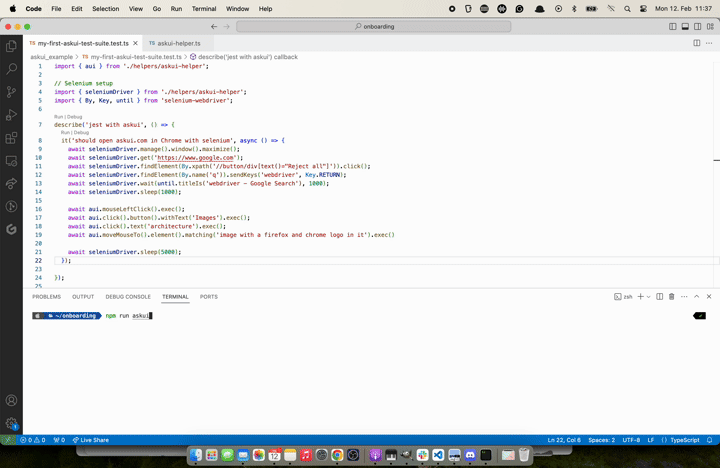
Conclusion
Integrating AskUI with a framework like Selenium comes down to configuring Selenium Webdriver in Jest. If you are solely automating in a web browser Selenium is a solid choice. Once you also want to manipulate a desktop app or interact with your operating system AskUI can take over.
Combining Selenium with AskUI gives you all the options to automate workflows over multiple applications.