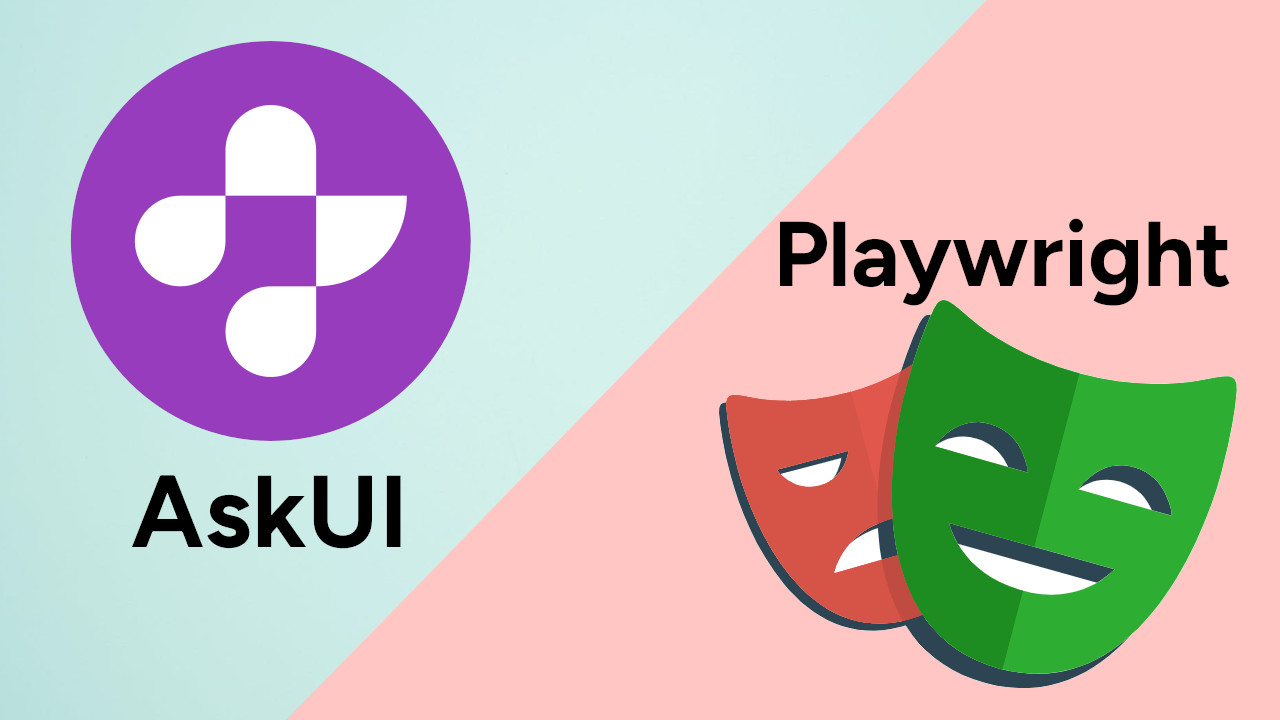
Playwright is the de facto standard when it comes to reliably testing browser applications across browsers and operating systems.
But what if your workflow under test leaves your browser and requires you to automate on a desktop application?
AskUI is not confined to a specific application because it uses visual selectors and real human-user-like input with keypresses and natural mouse movement. This makes it an ideal tool to accompany Playwright.
In this post you will learn to setup Playwright together with AskUI to define workflows that can leave the browser.
Prerequisites
Install Playwright
Playwright for JavaScript/TypeScript consists of two components.
1. The playwright node package
2. A browser installed through Playwright library
First you have to install playwright as a dev-dependency. Execute the following command in your terminal:
For this blog you will use Chromium as a browser. The installation through `playwright` is done like this:
Configure Playwright
You will configure Playwright and the Chromium browser in the file helpers/askui-helper.ts, so it will work correctly together with Jest.
- Import the necessary classes from
playwright
and define the variables - Configure chromium to be not headless and also slow down its execution. Also we want a
Desktop Chrome
as we are on desktop. - Close the
BrowserContext
and theBrowser
after the execution finished. - Export the Context so you can use it in your workflow
Write a Workflow
With the BrowserContext set up, hop over to the file my-first-askui-test-seuite.test.ts and import it there. Add this to the start of the file:
Now you can implement a simple workflow:
- Open a new tab
- Navigate to https://google.com/
- Click away the cookie banner
- Fill the search field with Playwright framework
- Press Enter on keyboard
- Switch to image search by clicking on the Button architecture
- Move the mouse cursor to the element that has an image with a red and a green mask in it (Playwright logo description)
Testrun
To run this you need to run this command in your terminal:
The following gif shows the execution of the whole workflow:
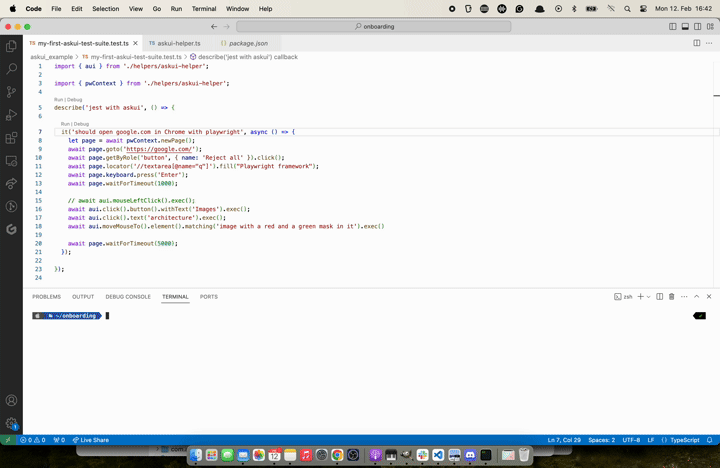
Conclusion
Combining AskUI with Playwright enhances automation capabilities to extend beyond web browsers. While Playwright is a solid choice for browser automation, AskUI takes the reins when dealing with desktop apps and operating systems.
This integration offers a practical toolkit for workflows across various applications for efficient automation.
More to explore
Get in touch
For media queries, drop us a message at info@askui.com