In this tutorial, we will automate web browser searching on Android devices. This tutorial assumes that you have already set up your Android test device, alongside the Android Development Environment. If you haven't set it up yet, you can check out our previous tutorial.
Requirements
- Android Studio installed
- Follow the setup in previous tutorial
1. Prepare the AskUI Test Environment
Install and Initialize AskUI
Please follow our descriptive tutorial (Windows, Linux, macOS).
Configure AskUI for Android Devices
We need to run the AskUI Controller manually with an extra argument to specify the runtime mode for Android.
# First find out the device id
adb devices
# Substitute <your-device-id> with
# the returned device id
AskUI-StartController -Runtime android -DeviceId "<your-device-id>"
2. Try Annotating
Make sure that your Android device is connected, or if you are using the Android Emulator, make sure that it is open and running on your computer.
AskUI provides a feature where you can monitor how the visible elements are detected by AskUI.
Try to change the code within askui_example/my-first-askui-test-suite.test.ts:
import { aui } from './helpers/askui-helper.ts';
describe('jest with askui', () => {
it('should show the annotation', async () => {
await aui.annotateInteractively();
});
});
and run,
AskUI-RunProject

💡 Annotation is Interactive
Try to hover your mouse on the red bounding box. It will let you know how to manipulate that element via AskUI Instructions.
3. Automate Web Searching
Now we are good to go for the actual automation process.
The automation consist of three steps:
- Open Chrome
- Select the search bar and type 'spacecraft'
- Click on the desired search result
1) Open Chrome
To open Chrome, we first have to figure out how we can let AskUI know where to click on.
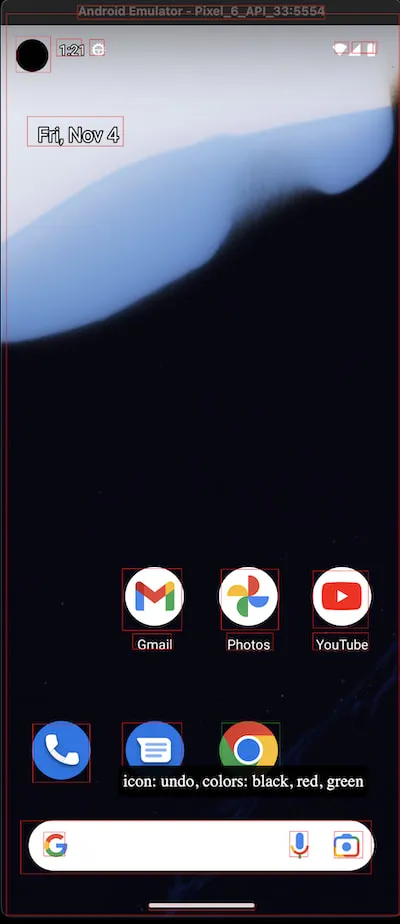
As we can see in the annotated information, the Chrome icon is recognized as an icon: undo. Indeed, we could also tell AskUI to select the icon: undo, but we will try to do it in a more precise way.
What we gonna do is:
(1) Select the search bar
(2) Type 'chrome'
(3) Select the icon above the text 'chrome'
This sideway approach will give us a more consistent result because typing chrome in the search bar will give us a more clearly understandable visual element.
Try to change your code according to this:
import { aui } from './helpers/askui-helper.ts';
describe('jest with askui', () => {
it('should open chrome', async () => {
// Here we try to avoid failing our test by using the try-catch phrase.
// It is because, 'textfield' and 'textarea' are seeming quite the same, even if they are tagged with different names.
try {
await aui.click().textfield().exec();
} catch (error) {
await aui.click().textarea().exec();
}
// Type the desired keyword into the search bar
await aui.type('chrome').exec();
// We wait for 1500 milliseconds, to make sure that
// the search result has been loaded before askui start to look for the search result.
await aui.waitFor(1500).exec();
// Then click the icon that is above the text 'chrome'
await aui.click().icon().above().text().withText('chrome').exec();
});
});
and run,
AskUI-RunProject
Now you will be able to see that Chrome has been opened.
2) Select the Search Bar and Type 'spacecraft'
Let's select the search bar of chrome, and type our desired keyword in there.

Add this code block to the bottom of our code:
// We first look for the search bar.
// Depending on the system language of your device,
// the default text within the search bar may differ.
await aui.click().text().withText('search or type web address').exec();
// Type our desired keyword and hit enter
await aui.type('spacecraft').exec();
await aui.pressAndroidKey('enter').exec();
In some cases, when searching in Google, you will be asked to give consent for the cookies.
To avoid our test from failing, we have to examine whether we got a pop-up for the cookie consent or not:
try {
// The `expect()` examines whether a specific element is detected or not.
// A command starting with `expect()` must always end with `exists()` or `notExists()`
await aui.expect().text().containsText('cookies').notExists().exec();
} catch (error) {
await aui.click().text().withText('read more').exec();
await aui.waitFor(1000).exec(); // wait until the scrolling animation has been finished
await aui.click().text().withText('accept all').exec();
}
// From here, we can write our next code
3) Click on the Desired Search Result
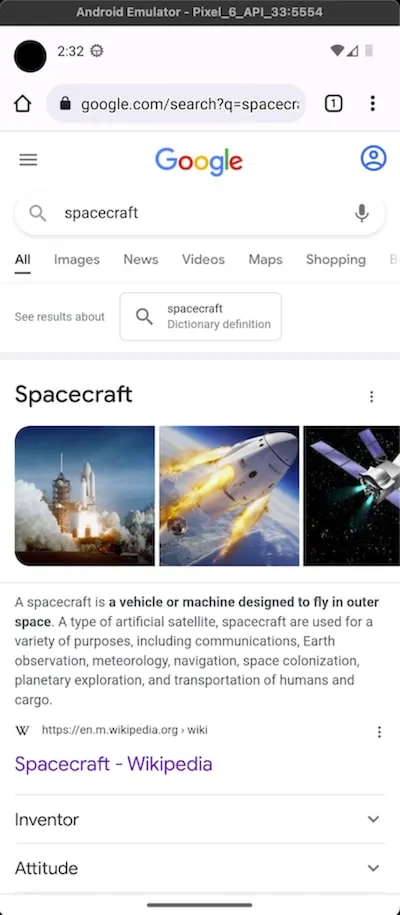
After clearing the cookie consent pop-up, we can see and click our desired search result. In our test case, we will look for the result from Wikipedia:
// We ask the askui to click the text that contains
// 'wikipedia' which is the text that is nearest to
// the text containing 'wikipedia.org'
await aui.click().text().containsText('wikipedia').nearestTo().text().containsText('wikipedia.org').exec();
Pay attention to the command nearestTo() that is interconnecting two different text elements.
AskUI offers several relational selectors, which enable you to select the desired element in an intuitively understandable way:
- above()
- below()
- contains()
- in()
- leftOf
- rightOf
- nearestTo
💡 About withText() and containsText()
You might wonder how withText() and containsText() differ. withText() tries to match the given text as the whole sequence, whereas containsText() tries to match the given text as a sub-text within the elements. Generally speaking, containsText() can be handier to match the text roughly, but you might face a test case where you want to find a specific text as a whole sequence.
4. Complete Test Code
import { aui } from './helpers/askui-helper.ts';
describe('jest with askui', () => {
it('should search spacecraft in chrome', async () => {
// Here we try to avoid failing our test by using the try-catch phrase.
// It is because, 'textfield' and 'textarea' are seeming quite the same, even if they are tagged with different names.
try {
await aui.click().textfield().exec();
} catch (error) {
await aui.click().textarea().exec();
}
// Type the desired keyword into the search bar
await aui.type('chrome').exec();
// We wait for 1500 miliseconds, to make sure that the search result has been loaded before askui start to look for the search result.
await aui.waitFor(1500).exec();
// Then click the icon that is above the text 'chrome'
await aui.click().icon().above().text().withText('chrome').exec();
// We wait the Chrome app to be launched
await aui.waitFor(1500).exec();
// We first look for the search bar. Depending on the system language of your device, the default text within the search bar may differ.
await aui.click().text().withText('search or type web address').exec();
// Type our desired keyword and hit enter
await aui.type('spacecraft').exec();
await aui.pressAndroidKey('enter');
// We wait for the search result to be loaded
await aui.waitFor(3000).exec();
try {
// The `expect()` examines whether a specific element is detected or not.
// A command starting with `expect()` must always end with `exists()` or `notExists()`
await aui.expect().text().containsText('cookies').notExists().exec();
} catch (error) {
await aui.click().text().withText('read more').exec();
await aui.waitFor(1000).exec(); // wait until the scrolling animation has been finished
await aui.click().text().withText('accept all').exec();
}
// We ask the askui to click the text that contains 'wikipedia' which is the text that is nearest to the text containing 'wikipedia.org'
await aui.click().text().containsText('wikipedia').nearestTo().text().containsText('wikipedia.org').exec();
});
});
5. Done
We have covered a use case of AskUI to automate web searching in Android devices.