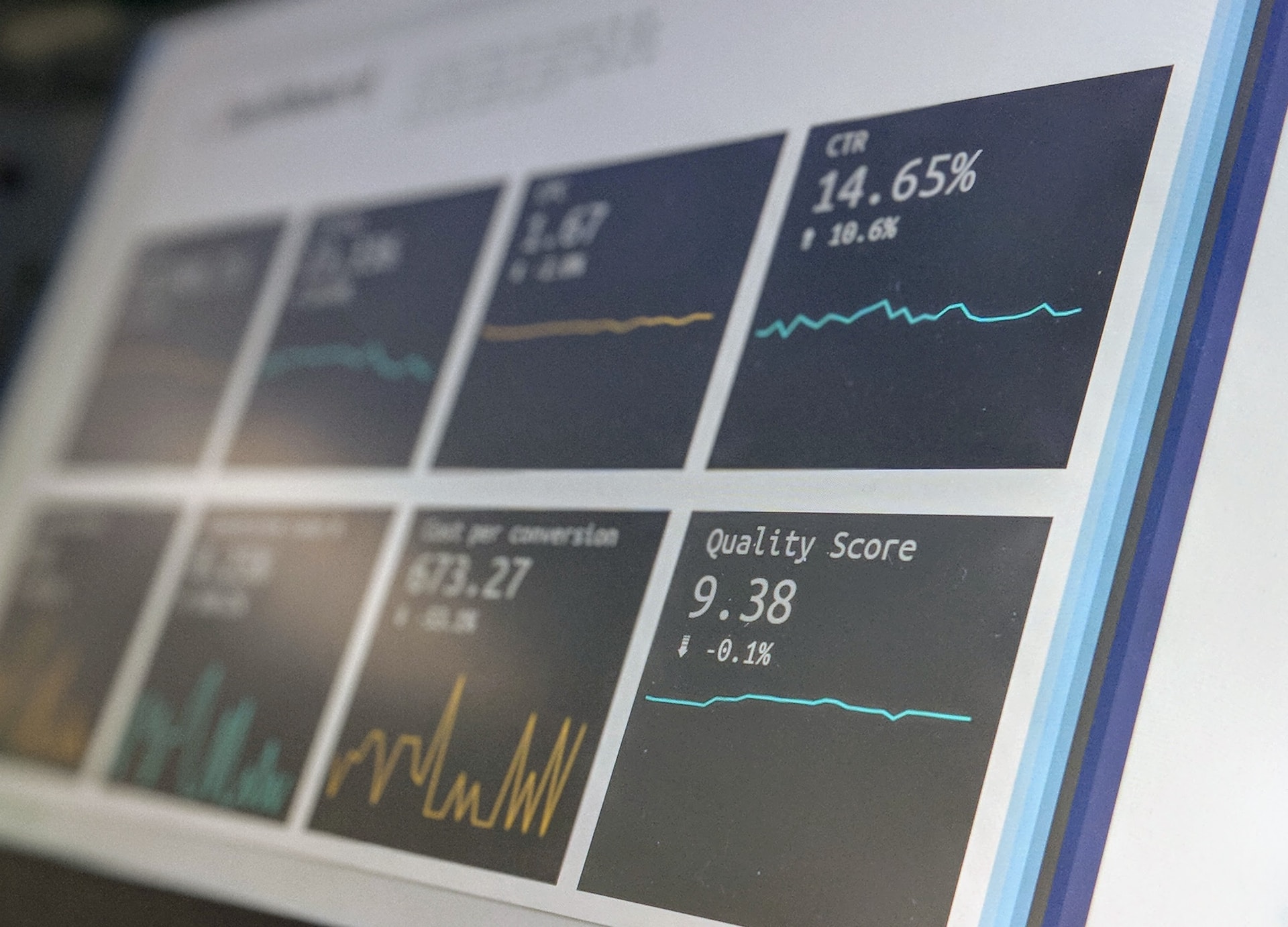
Debugging UI-Automations can be a real pain especially when you run them inside a pipeline with no chance to observe what is actually happening during execution.
Wouldn't it be nice to get a report of failed automation steps that include screenshot before and after an execution and also a video?
We've recently added video recording support to our UI controller, enabling you to record a video for each step you execute and add it to a report of your AskUI runs.
We also provide an interface you can implement so you can integrate it into your favorite reporting tool like for example Allure.
Implementing A Reporter
To help you get started, we've created an example repository for Allure and also a repository where we collect reporter implementations.
Implementing your own reporter involves two main steps:
- Implementing the reporter interface
- Configuring the reporter within the askui-helper.ts.
Depending on your chosen reporter framework, you may not need to implement every function in the interface.
For instance, our Allure reporter only includes the config and onStepEnd() functions.
Optionally, you can provide mappings for the steps of __Jest__ to your reporter framework, tailoring the integration to your preferences.
To integrate video recording into your reporting tool, configure your reporter in the askui-helper.ts file within the UIControlClient. Additionally, extract the recorded video from the UIController and add it to your reporter.
Here's an example of how that is done:
Allure Example
Our Allure example repository also publishes its report to a website: Check it out here!
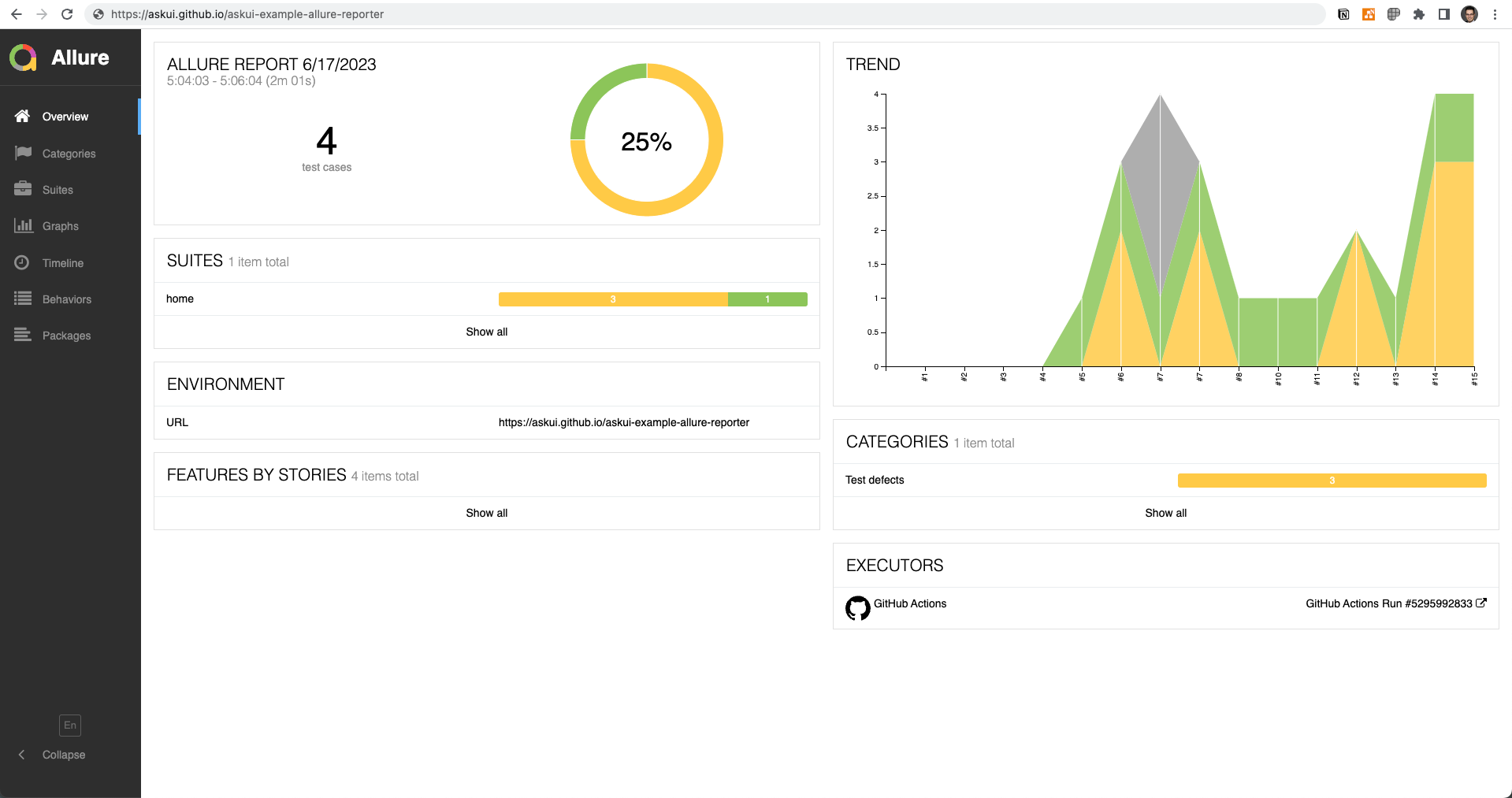
.
Links for Already Implemented Reporters
We've created a community repository on our GitHub space where you can find and contribute implemented reporters. If you need inspiration or want to share your custom reporter with the community this is the right place to start!
All reporters and Instructions to implement your own
Conclusion
Incorporating screenshots and videos into your executed steps reports can significantly enhance your reports, as demonstrated in the provided example.
If a specific reporter is not implemented already, you can implement it on your own and integrate it with the instructions and examples given in our repositories.
If you need help, please join our Discord where the AskUI staff and the community are ready to help you out!
More to explore
Get in touch
For media queries, drop us a message at info@askui.com